Understanding the C ANSI Keyboard Decoder Function: A Complete Guide
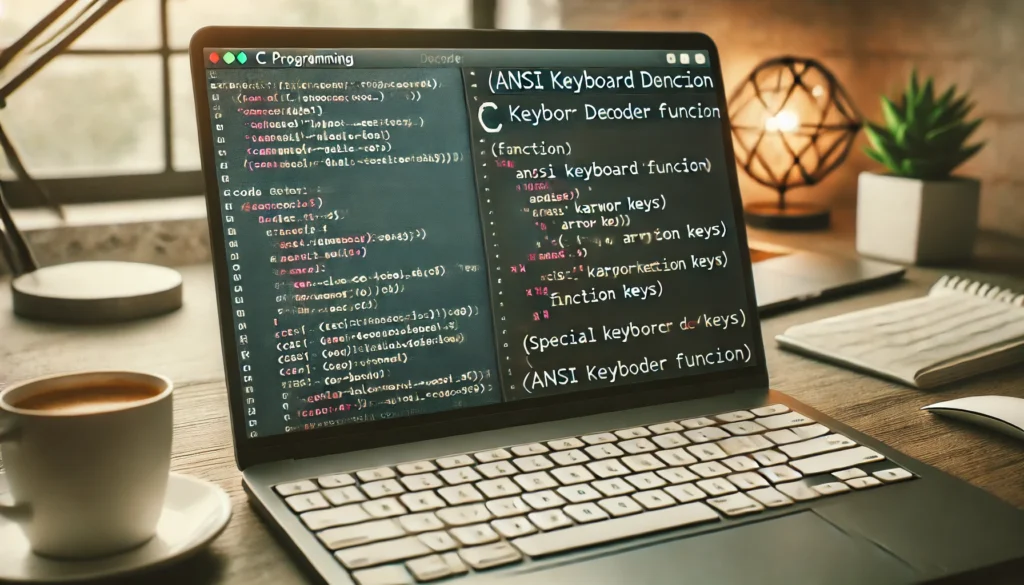
Introduction to the C ANSI Keyboard Decoder Function
The C ANSI Keyboard Decoder Function is a vital component in C programming used to decode keyboard input, converting raw key presses into usable characters or control commands. This functionality is particularly important when dealing with special keys like the arrow keys, function keys (F1-F12), and other non-alphanumeric keys. It ensures that the input from these special keys is processed in a way that makes sense to the program, whether for text processing or interactive applications.
In this guide, we’ll explore the C ANSI Keyboard Decoder Function in detail, covering its importance, the underlying mechanics of how it works, practical examples, and advanced usage scenarios. By the end of this article, you’ll have a comprehensive understanding of how to implement and optimize the function in your C projects.
Understanding Keyboards and Scan Codes
The Role of Scan Codes in Keyboard Input
When a key is pressed on a keyboard, it doesn’t directly send a character or function to the computer. Instead, it sends a scan code — a unique number assigned to each key. These scan codes are used by the computer to identify which key has been pressed, and then the system processes this scan code accordingly. The process involves translating this raw scan code into a readable character or action. The C ANSI Keyboard Decoder Function is the mechanism that decodes these scan codes into appropriate values that the program can interpret.
Types of Scan Codes
There are two main types of scan codes that a keyboard can generate:
- Basic Scan Codes: These correspond to standard alphanumeric keys (A-Z, 0-9), punctuation marks, and other standard characters.
- Extended Scan Codes: These correspond to special keys, such as function keys (F1, F2, etc.), arrow keys, and control keys like the Enter or Esc key.
The C ANSI Keyboard Decoder Function ensures that all types of scan codes are correctly translated and handled in the C program.
Overview of the ANSI Standard
The ANSI standard is a set of character encoding rules that were developed by the American National Standards Institute. In the context of keyboard input, ANSI codes refer to a standard set of character codes used to represent keys pressed on a keyboard, particularly in text-based terminal systems.
The ANSI standard also includes escape sequences, which are used to control various terminal operations like cursor positioning, text color, and screen clearing. While the C ANSI Keyboard Decoder Function primarily focuses on mapping scan codes to character codes, understanding the broader ANSI standard is crucial for dealing with more complex control sequences.
Decoding Scan Codes to Characters
The Basics of Decoding Keyboard Input in C
Decoding key presses in C involves reading the raw scan codes and translating them into usable characters or control commands. In this section, we will go through the process of how the C ANSI Keyboard Decoder Function works.
When a key is pressed, the corresponding scan code is passed to the C program. The C ANSI Keyboard Decoder Function then looks up this scan code in a predefined table or list, which maps scan codes to their corresponding ANSI values or ASCII codes.
For instance:
- The scan code for the “A” key might correspond to the ASCII value of 65.
- The scan code for the “Enter” key could correspond to a special control code that moves the cursor or executes a command.
Handling Special Keys
Handling special keys such as function keys (F1-F12), arrow keys, and other control keys is an essential feature of the C ANSI Keyboard Decoder Function. For example, the scan code for the right arrow key is different from the one for the “A” key. The decoder needs to map these keys to specific functions or actions, such as cursor movement or menu navigation.
Key Functions and Methods in C ANSI Keyboard Decoding
Basic Key Decoding Functions in C
In C programming, the basic function to handle keyboard input often involves the use of getchar() or getch() functions. These functions read a single key press and return the corresponding character or key code. To decode special keys, additional logic is implemented, usually by mapping scan codes to their ANSI or ASCII equivalents.
Example code for reading a key in C:
#include <stdio.h>
int main() {
char ch;
printf(“Press a key: “);
ch = getchar();
printf(“You pressed: %c\n”, ch);
return 0;
}
This simple program reads a key from the user and outputs the character corresponding to that key. However, for decoding special keys like the Arrow Keys or Function Keys, more advanced techniques are required.
Handling Modifier Keys (Shift, Ctrl, Alt)
Modifier keys such as Shift, Ctrl, and Alt require special handling because they change the behavior of other keys. For instance, pressing Shift + A generates an uppercase “A”, while pressing Ctrl + C sends a control signal to terminate a program. The C ANSI Keyboard Decoder Function ensures that combinations like these are decoded appropriately, often by checking if any modifier keys are pressed in conjunction with a regular key.
Extended Key Support
Extended keys, like F1 through F12 or Home, End, Page Up, and Page Down, require additional handling since they produce a range of non-printable scan codes. The C ANSI Decoder Function maps these scan codes to specific actions in the program.
How the ANSI Decoder Function Works in Real-Time
In real-time applications, such as text-based games or interactive systems, the C ANSI Keyboard Decoder Function must work efficiently to ensure smooth user interaction. Here’s an example of how the decoder processes a key press:
- The user presses a key on the keyboard.
- The scan code for that key is sent to the program.
- The C ANSI Decoder Function checks the scan code against a map of known codes and decodes it into a character or control code.
- The decoded value is then passed to the application to trigger the corresponding action.
Example code snippet for real-time input handling:
#include <conio.h>
int main() {
int key;
while(1) {
key = getch();
if(key == 224) { // Special key detected
key = getch(); // Get the actual special key (e.g., arrow key)
// Process the special key
}
else {
printf(“You pressed: %c\n”, key);
}
}
return 0;
}
Error Handling and Debugging
Common Errors in ANSI Keyboard Decoding
When decoding keyboard inputs, common issues may arise due to incorrect mapping of scan codes or unsupported key combinations. For instance, a scan code might not match any predefined key, leading to incorrect behavior.
To debug this, developers can use logging or print statements to track the scan codes and identify mismatches. Furthermore, proper error handling can be implemented to ensure that invalid or unrecognized scan codes are ignored, preventing the program from crashing.
Conclusion
In conclusion, the C ANSI Keyboard Decoder Function plays a crucial role in handling keyboard input, especially when dealing with non-standard keys like function keys and special characters. By decoding scan codes into readable characters or control commands, it enables developers to create interactive, user-friendly applications. Whether you’re building a game, a text-based terminal application, or a system with complex keyboard input requirements, understanding how to effectively implement and troubleshoot the C ANSI Keyboard Decoder Function is essential.
Frequently Asked Questions (FAQs)
What is the difference between scan codes and ASCII codes?
Scan codes are raw data sent from the keyboard representing a physical key press, while ASCII codes represent characters or control commands used in text encoding.
How can I detect multiple key presses at once in C?
Use the kbhit() function from the conio.h library to check if multiple keys are pressed, or implement custom logic to handle key combinations.
Can I customize the C ANSI Decoder for a non-standard keyboard layout?
Yes, you can modify the scan code mappings to support custom keyboard layouts or special configurations.
How do I handle non-printable keys like Escape or Tab?
The ANSI Decoder can be extended to handle non-printable keys by mapping their scan codes to predefined control codes.
Is the C ANSI Decoder compatible with all operating systems?
The C ANSI Decoder function is primarily platform-dependent. Ensure that the input handling functions (getch(), getchar(), etc.) are compatible with the OS you are developing for.
Recommended Articles:
Dwayne Khallique: A Visionary in AI, Machine Learning, and Technology Innovation
CS Nite-Beam Spotlightlight Model 901: The Complete Guide
Visore per Vedere TV a 100 Pollici: The Future of Personal Entertainment
How Often to Water Golden Raisin Tree in Albuquerque: A Comprehensive Guide
Understanding the C ANSI Keyboard Decoder Function: A Complete Guide
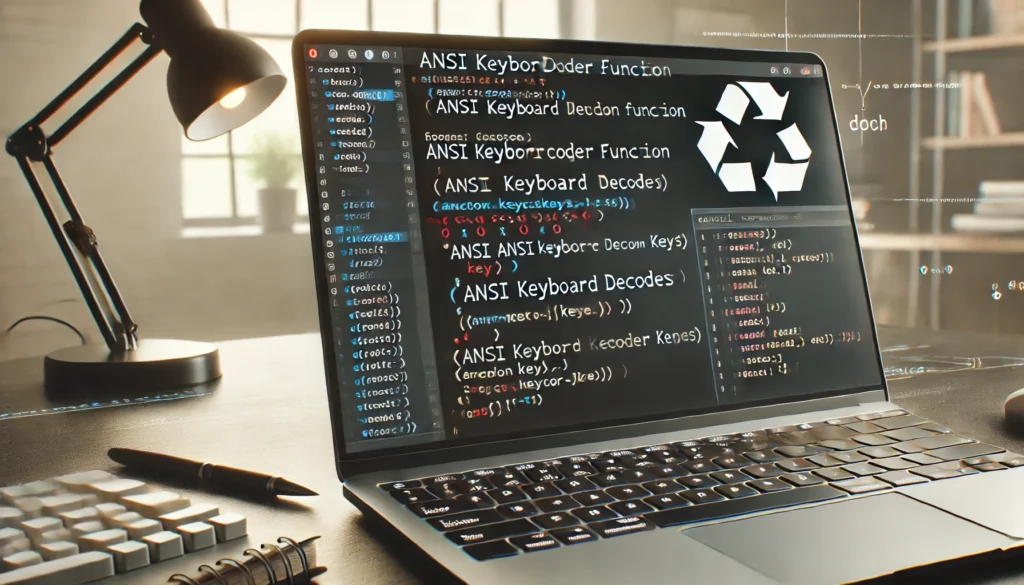
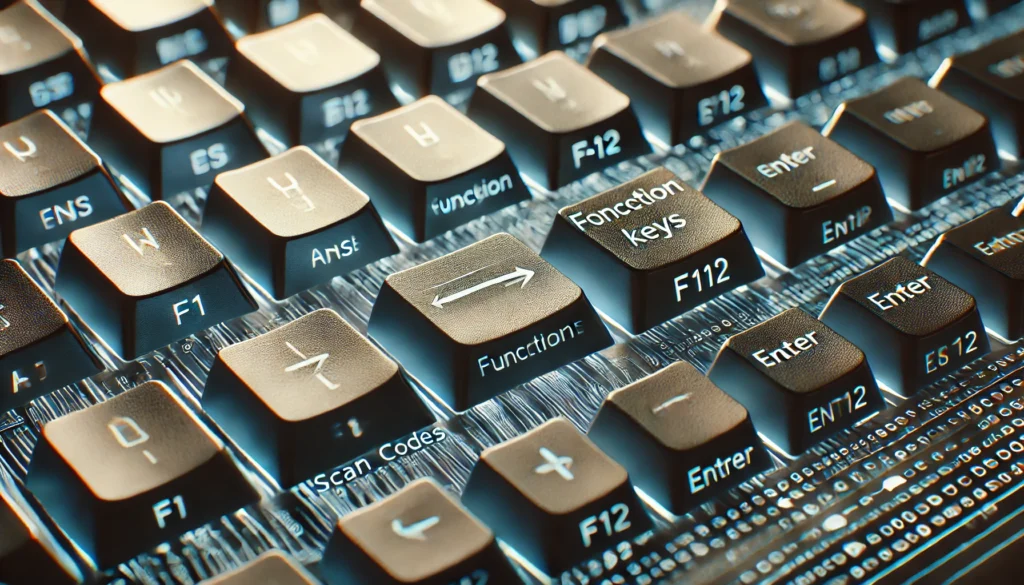
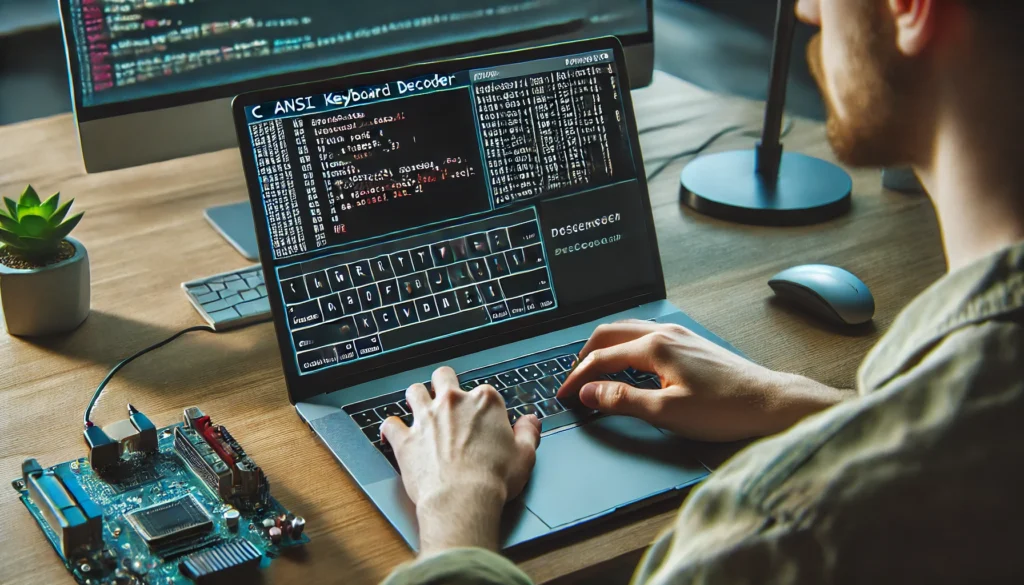